此程序为一个学生学籍管理系统,用户可以在程序开始运行时选择学生人数和课程人数,在输入之后便可进行操作, 计算每科的总成绩、平均成绩,计算每个人的总成绩、平均成绩,按分数排序,按学号排序,通过学号搜索信息,通过姓名搜索信息,各科成绩分析,列出所有人员信息 等功能。 2.功能模块图 - . 选择学生人数和课程人数。
- . 计算每科的总成绩、平均成绩
- . 计算每个人的总成绩、平均成绩
- . 按分数排序
- .按学号排序
- . 通过学号搜索信息
- . 通过姓名搜索信息
- . 各科成绩分析
- . 列出所有人员信息
2.详细流程 首先定义结构体存放学生的基本属性 //结构体的定义,表示每个学生的信息 typedef struct //定义了一个名字叫做student的结构体 { int school_number; //学号 char name[128]; //姓名 float object[128]; //科目 float total_sort; //总成绩 float average_sort; //平均成绩 }student; 接着定义了四个全局变量 //定义了四个全局变量 int how_many_people; //多少人 int how_many_object; //多少科 student theClass[30],temp; //结构体数组,表示多少个人,30可以换,temp交换用的一个结构体 char anything; //记录任意键的变量名 接着定义十个函数分别完成各个的功能,每个参数引入的形式参数 void how_many_people_and_object(); //一共几人几科 void input_record_manually(); //输入信息依次输入学生名字以及课程科目平均成绩 void Calculate_total_and_average_score_of_every_course(); //计算每科的总成绩平均成绩 运用两个for循环统计每个科目的每个人的信息相加求出总和,除以人数求出平均值 void Calculate_total_and_average_score_of_every_student(); //计算每个人的总成绩平均成绩,由结构体直接读出来 voidSort_in_descending_order_by_total_score_of_every_student();//按总成绩排名 用冒泡排序 将第一个元素与之后的n个元素比较,如果有比第一个元素大的元素则将其置换,继续比较,则一次比较完毕,最后一个元素是最大的,再将第二个元素与n-1个元素比较重复过程。 void Sort_in_ascending_order_by_number(); //按学号大小排名用冒泡排序 将第一个元素与之后的n个元素比较,如果有比第一个元素大的元素则将其置换,继续比较,则一次比较完毕,最后一个元素是最大的,再将第二个元素与n-1个元素比较重复过程。 void Search_by_number();//通过学号来搜索 void Search_by_name();//通过姓名来搜索 void Statistic_analysis_for_every_course();//分析各科成绩 void List_record();//列出信息 流程图: 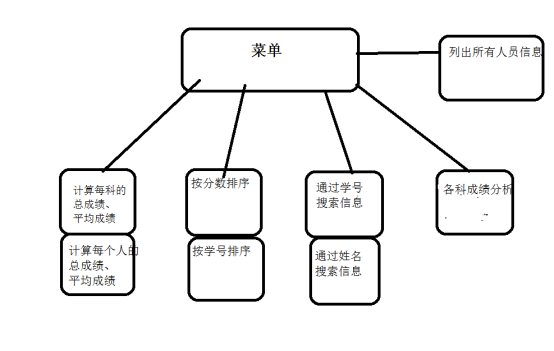
- #include<stdio.h> //C语言的头文件
- #include<windows.h> //windows的头文件
- #include<conio.h> //控制台输入输出头文件
- #include<time.h> //时间的头文件
- void how_many_people_and_object(); //一共几人几科
- void input_record_manually(); //输入信息
- void Calculate_total_and_average_score_of_every_course(); //计算每科的总成绩平均成绩
- void Calculate_total_and_average_score_of_every_student(); //计算每个人的总成绩平均成绩
- void Sort_in_descending_order_by_total_score_of_every_student();//按总成绩排名
- void Sort_in_ascending_order_by_number(); //按学号大小排名
- void Search_by_number(); //通过学号来搜索
- void Search_by_name(); //通过姓名来搜索
- void Statistic_analysis_for_every_course(); //分析各科成绩
- void List_record(); //列出信息
-
- //结构体的定义,表示每个学生的信息
- typedef struct //定义了一个名字叫做student的结构体
- {
- int school_number; //学号
- char name[128]; //姓名
- float object[128]; //科目
- float total_sort; //总成绩
- float average_sort; //平均成绩
- }student;
-
- //定义了四个全局变量
- int how_many_people; //多少人
- int how_many_object; //多少科
- student theClass[30],temp; //结构体数组,表示多少个人,30可以换,temp交换用的一个结构体
- char anything; //记录任意键的变量名
-
- //函数的定义
- void how_many_people_and_object()
- {
- printf("请输入学生人数:");
- scanf("%d",&how_many_people);
- printf("请输入总科目:");
- scanf("%d",&how_many_object);
- }
- void input_record_manually()
- {
- char ch;
- for(int i=0;i<how_many_people;i++)
- {
- printf("请输入第 %d 个人的姓名:",i+1);
- scanf("%s",&theClass[i].name);
-
- printf("请输入第 %d 个人的学号:",i+1);
- scanf("%d",&theClass[i].school_number);
-
- float sum=0;
- for(int j=0;j<how_many_object;j++)
- {
- printf("请输入第 %d 个人的第 %d 科成绩:",i+1,j+1);
- scanf("%f",&theClass[i].object[j]);
-
- sum=sum+theClass[i].object[j];
- theClass[i].total_sort=sum;
- theClass[i].average_sort=theClass[i].total_sort/how_many_object;
- }
- printf("总成绩: %.2f\n",theClass[i].total_sort);
- printf("平均成绩: %.2f\n",theClass[i].average_sort);
- }
- printf("任意键返回主菜单");
- ch=getche();
- }
- void Calculate_total_and_average_score_of_every_course()
- {
- char ch;
- float total_sum=0;
- float average_sum=0;
- for(int j=0;j<how_many_object;j++)
- {
- for(int i=0;i<how_many_people;i++)
- {
- total_sum = theClass[i].object[j] + total_sum;
- average_sum = total_sum / how_many_people;
- }
- printf("第 %d 科的总成绩:%.2f 平均成绩:%.2f\n",j+1,total_sum,average_sum);
- total_sum=0;
- average_sum=0;
- }
-
- printf("任意键返回主菜单");
- ch=getche();
- }
- void Calculate_total_and_average_score_of_every_student()
- {
- char ch;
- for(int i=0;i<how_many_people;i++)
- {
- printf("%s 的总成绩: %.2f 平均成绩: %.2f\n",theClass[i].name,theClass[i].total_sort,theClass[i].average_sort);
- }
- printf("任意键返回主菜单");
- ch=getche();
- }
- void Sort_in_descending_order_by_total_score_of_every_student()
- {
- char ch;
- for(int i=0;i<how_many_people;i++) //冒泡排序
- {
- for(int j=i+1;j<how_many_people;j++)
- {
- if(theClass[i].total_sort<theClass[j].total_sort)
- {
- temp=theClass[i];
- theClass[i]=theClass[j];
- theClass[j]=temp;
- }
- }
- }
- for(int ii=0;ii<how_many_people;ii++)
- {
- printf("第%d名:%s--%.2f\n",ii+1,theClass[ii].name,theClass[ii].total_sort);
- }
- printf("任意键返回主菜单");
- ch=getche();
- }
- void Sort_in_ascending_order_by_number()
- {
- char ch;
- for(int i=0;i<how_many_people;i++)
- {
- for(int j=i+1;j<how_many_people;j++)
- {
- if(theClass[i].school_number>theClass[j].school_number)
- {
- temp=theClass[i];
- theClass[i]=theClass[j];
- theClass[j]=temp;
- }
- }
- }
- for(int ii=0;ii<how_many_people;ii++)
- {
- printf("%d--%s--%.2f\n",theClass[ii].school_number,theClass[ii].name,theClass[ii].total_sort);
- }
- printf("任意键返回主菜单");
- ch=getche();
- }
- void Search_by_number()
- {
- char ch;
- int number;
- printf("请输入学号:");
- scanf("%d",&number);
- system("cls");
- for(int i=0;i<how_many_people;i++)
- {
- if(number - theClass[i].school_number == 0)
- {
- printf("姓名:%s\n",theClass[i].name);
- printf("学号:%d\n",theClass[i].school_number);
- for(int j=0;j<how_many_object;j++)
- {
- printf("第 %d 科成绩:%.2f\n",j+1,theClass[i].object[j]);
- }
- printf("总成绩:%.2f\n平均成绩:%.2f\n\n",theClass[i].total_sort,theClass[i].average_sort);
- }
- }
- printf("任意键返回主菜单");
- ch=getche();
- }
- void Search_by_name()
- {
- char ch;
- char n[128];
- printf("请输入姓名:");
- scanf("%s",&n);
- for(int i=0;i<how_many_people;i++)
- {
- if(strcmp(n,theClass[i].name)==0)
- {
- printf("姓名:%s\n",theClass[i].name);
- printf("学号:%d\n",theClass[i].school_number);
- for(int j=0;j<how_many_object;j++)
- {
- printf("第 %d科成绩:%.2f\n",j+1,theClass[i].object[j]);
- }
- printf("总成绩:%.2f\n平均成绩:%.2f\n",theClass[i].total_sort,theClass[i].average_sort);
- }
- }
- printf("任意键返回主菜单");
- ch=getche();
- }
- void Statistic_analysis_for_every_course()
- {
- char ch;
- float a[6][6]={0}; //第几科的第几类成绩(优良中差及不及),最大为6课,可以改
- for(int i=0;i<how_many_people;i++)
- {
- for(int j=0;j<how_many_object;j++)
- {
- if(theClass[i].object[j]>=90 && theClass[i].object[j]<=100)
- a[j][0]++;
- else if(theClass[i].object[j]>=80 && theClass[i].object[j]<=89)
- a[j][1]++;
- else if(theClass[i].object[j]>=70 && theClass[i].object[j]<=79)
- a[j][2]++;
- else if(theClass[i].object[j]>=60 && theClass[i].object[j]<=69)
- a[j][3]++;
- else
- a[j][4]++;
- }
- }
- for(int j=0;j<how_many_object;j++)
- {
- printf("第 %d 科优秀的人数是:%.2f 所占百分比是:%.0f %%\n",j+1,a[j][0],a[j][0]/how_many_people*100);
- printf("第 %d 科良好的人数是:%.2f 所占百分比是:%.0f %%\n",j+1,a[j][1],a[j][1]/how_many_people*100);
- printf("第 %d 科中等的人数是:%.2f 所占百分比是:%.0f %%\n",j+1,a[j][2],a[j][2]/how_many_people*100);
- printf("第 %d 科及格的人数是:%.2f 所占百分比是:%.0f %%\n",j+1,a[j][3],a[j][3]/how_many_people*100);
- printf("第 %d 科不及的人数是:%.2f 所占百分比是:%.0f %%\n\n",j+1,a[j][4],a[j][4]/how_many_people*100);
- }
- printf("任意键返回主菜单");
- ch=getche();
- }
- void List_record()
- {
- char ch;
- for(int i=0;i<how_many_people;i++)
- {
- printf("%s\n",theClass[i].name);
- printf("%d号\n",theClass[i].school_number);
-
- for(int j=0;j<how_many_object;j++)
- {
- printf("第 %d 科成绩:%.2f\n",j+1,theClass[i].object[j]);
- }
- printf("总成绩:%.2f\n平均成绩:%.2f\n\n",theClass[i].total_sort,theClass[i].average_sort);
- }
- printf("任意键返回主菜单");
- ch=getche();
- }
-
- //主函数
- int main()
- {
- int choise;
-
-
- printf("学生成绩管理系统\n\n");
- how_many_people_and_object();
-
- part:
- system("cls");
- printf("1.Input record manually\n");
- printf("2.Calculate total and average score of every course\n");
- printf("3.Calculate total and average score of every student\n");
- printf("4.Sort in descending order by total score of every student\n");
- printf("5.Sort in ascending order by number\n");
- printf("6.Search by number\n");
- printf("7.Search by name\n");
- printf("8.Statistic analysis for every course\n");
- printf("9.List record\n");
- printf("0.Exit\n");
- printf("Please input your choice:");
- scanf("%d",&choise);
- if(choise==1)
- {
- system("cls");
- input_record_manually();
- goto part;
- }
- else if(choise==2)
- {
- system("cls");
- Calculate_total_and_average_score_of_every_course();
- goto part;
- }
- else if(choise==3)
- {
- system("cls");
- Calculate_total_and_average_score_of_every_student();
- goto part;
- }
- else if(choise==4)
- {
- system("cls");
- Sort_in_descending_order_by_total_score_of_every_student();
- goto part;
- }
- else if(choise==5)
- {
- system("cls");
- Sort_in_ascending_order_by_number();
- goto part;
- }
- else if(choise==6)
- {
- system("cls");
- Search_by_number();
- goto part;
- }
- else if(choise==7)
- {
- system("cls");
- Search_by_name();
- goto part;
- }
- else if(choise==8)
- {
- system("cls");
- Statistic_analysis_for_every_course();
- goto part;
- }
- else if(choise==9)
- {
- system("cls");
- List_record();
- goto part;
- }
- else if(choise==0)
- return 0;
- else
- ;
- return 0;
- }
复制代码
4.技术关键和设计心得
在这次课程设计中,我们首先对系统的整体功能进行了构思,然后用结构化分析方法进行分析,将整个系统清楚的划分为几个模块,再根据每个模块的功能编写代码。而且尽可能的将模块细分,最后在进行函数的调用。我们在函数的编写过程中,我们不仅用到了for循环、while循环和switch语句,还用到了函数之间的调用(包括递归调用)。由于我们是分工编写代码,最后需要将每个人的代码放到一起进行调试。因为我们每个人写的函数的思想不都一样,所以在调试的过程中也遇到了困难,但经过我们耐心的修改,终于功夫不负有心人,我们成功了!
完整的Word格式文档51黑下载地址:
学生学籍管理系统 - 副本.docx
(56.68 KB, 下载次数: 9)
|