工作后很多东西都是现用现学,不用就忘,如果不记录一下估计有一天就归零了。
从没系统的学过android,都是偶尔看看书写点Demo。最近项目上可能需要用到手持设备,所以复习一下。项目中设计到文件上传的功能,这样文件资源浏览器是必不可少的控件。先百度了一下找到一位CSDN猴子的代码(http://blog.csdn.net/x605940745/article/details/12580367)照着模仿下基本的功能出来了。但是有些不完善的地方。看他的layout与后台代码的ID都没有对应上,想必这两段的代码非出自一个Demo吧!
首先说第一个问题:加载出来的子文件夹单击没有相应。实现使用ListView加载更多的xml布局。所有的触发事件都有,加断点就是不进啊。。。百度查了一下,后来找到了原因。问题出在ListView子控件上有ImageButton元素,这种情况下单击ListView时子控件会首先获得focus。所以导致单击ListView无效。解决办法吧ImageButton换成ImageView就OK了
第二个问题:只有返回home没有返回上级菜单。这个比较简单,只需要用File.getParentFile();就OK了,但要注意的是判断是否为跟节点,因根节点在获取夫级元素就会抛异常。
- Layout(activity_documents_browser.xml)源码:
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- xmlns:tools="http://schemas.android.com/tools"
- android:layout_width="match_parent"
- android:layout_height="match_parent"
- android:layout_gravity="center_horizontal"
- android:background="#000000"
- android:orientation="vertical"
- tools:context="com.tonfun.smapp.DocumentsBrowserActivity" >
- <LinearLayout
- android:layout_width="wrap_content"
- android:layout_height="32dp" >
- <ImageView
- android:id="@+id/imageBt1"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:focusable="false"
- android:padding="3dp"
- android:src="@drawable/img_back" />
- <TextView
- android:id="@+id/txt1"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:focusable="false"
- android:padding="3dp"
- android:textColor="#fff" />
- </LinearLayout>
- <View
- android:layout_width="fill_parent"
- android:layout_height="3dp"
- android:background="#218fff" />
- <ListView
- android:id="@+id/listFile"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:descendantFocusability="blocksDescendants" >
- </ListView>
- </LinearLayout>
- Layout(activity_folder.xml)源码
- <?xml version="1.0" encoding="utf-8"?>
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:orientation="vertical" >
- <LinearLayout
- android:layout_width="fill_parent"
- android:layout_height="30dp" >
- <ImageView
- android:id="@+id/imageBt1"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:focusable="false"
- android:padding="2dp"
- android:src="@drawable/img_home" />
- <TextView
- android:id="@+id/txt1"
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:focusable="false"
- android:padding="2dp"
- android:textColor="#fff" />
- </LinearLayout>
- <View
- android:layout_width="fill_parent"
- android:layout_height="1dp"
- android:background="#fff" />
- </LinearLayout>
- 后台(JAVA)代码 :
- package com.tonfun.smapp;
- import java.io.File;
- import java.util.ArrayList;
- import java.util.HashMap;
- import java.util.List;
- import java.util.Map;
- import android.app.Activity;
- import android.os.Bundle;
- import android.os.Environment;
- import android.provider.MediaStore.Images;
- import android.view.Menu;
- import android.view.MenuItem;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.AdapterView;
- import android.widget.AdapterView.OnItemClickListener;
- import android.widget.ImageButton;
- import android.widget.ImageView;
- import android.widget.ListView;
- import android.widget.SimpleAdapter;
- import android.widget.TextView;
- import android.widget.Toast;
- public class DocumentsBrowserActivity extends Activity {
- private ListView listfile;
- // 当前文件目录
- private String currentpath;
- private TextView txt1;
- private ImageView images;
- private TextView textview;
- private ImageView imagebt1;
- private File fBeforePath;
- private int[] img = { R.drawable.img_files, R.drawable.img_folder,
- R.drawable.img_home };
- private File[] files;
- private SimpleAdapter simple;
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_documents_browser);
- listfile = (ListView) findViewById(R.id.listFile);
- txt1 = (TextView) findViewById(R.id.txt1);
- imagebt1 = (ImageView) findViewById(R.id.imageBt1);
- init(Environment.getExternalStorageDirectory());
- fBeforePath = Environment.getExternalStorageDirectory().getParentFile();
- listfile.setOnItemClickListener(new OnItemClickListener() {
- @Override
- public void onItemClick(AdapterView<?> arg0, View arg1, int arg2,
- long arg3) {
- // TODO Auto-generated method stub
- // 获取单击的文件或文件夹的名称
- String folder = ((TextView) arg1.findViewById(R.id.txt1))
- .getText().toString();
- try {
- File filef = new File(currentpath + '/' + folder);
- if (!filef.isFile()) {
- init(filef);
- } else {
- if (filef.getName().lastIndexOf('.') >= 0) {
- Toast.makeText(
- DocumentsBrowserActivity.this,
- "扩展名:"
- + filef.getName().substring(
- filef.getName()
- .lastIndexOf('.'),
- filef.getName().length())
- + ";绝对路径:"
- + filef.getAbsolutePath(),
- Toast.LENGTH_SHORT).show();
- }
- }
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
- });
- // 回根目录
- imagebt1.setOnClickListener(new OnClickListener() {
- @Override
- public void onClick(View v) {
- // init(Environment.getExternalStorageDirectory());
- if (!fBeforePath.getAbsolutePath().equals("/")) {
- init(fBeforePath);
- } else {
- Toast.makeText(DocumentsBrowserActivity.this, "提示:已为顶级目录!",
- Toast.LENGTH_SHORT).show();
- }
- }
- });
- }
- // 界面初始化
- public void init(File f) {
- if (Environment.getExternalStorageState().equals(
- Environment.MEDIA_MOUNTED)) {
- // 获取SDcard目录下所有文件名
- fBeforePath = f.getParentFile();
- files = f.listFiles();
- if (!files.equals(null)) {
- currentpath = f.getPath();
- try {
- txt1.setText("当前目录为:" + f.getPath());
- } catch (Exception ex) {
- }
- List<Map<String, Object>> list = new ArrayList<Map<String, Object>>();
- for (int i = 0; i < files.length; i++) {
- Map<String, Object> maps = new HashMap<String, Object>();
- if (files[i].isFile())
- maps.put("image", img[0]);
- else
- maps.put("image", img[1]);
- maps.put("filenames", files[i].getName());
- list.add(maps);
- }
- simple = new SimpleAdapter(this, list,
- R.layout.activity_folder, new String[] { "image",
- "filenames" }, new int[] { R.id.imageBt1,
- R.id.txt1 });
- listfile.setAdapter(simple);
- }
- } else {
- System.out.println("该文件为空");
- }
- }
- }
复制代码
不贴下载连接了,着急会宿舍睡觉 (~﹃~)~zZ
忘记贴效果图了(/ □ \)。。。
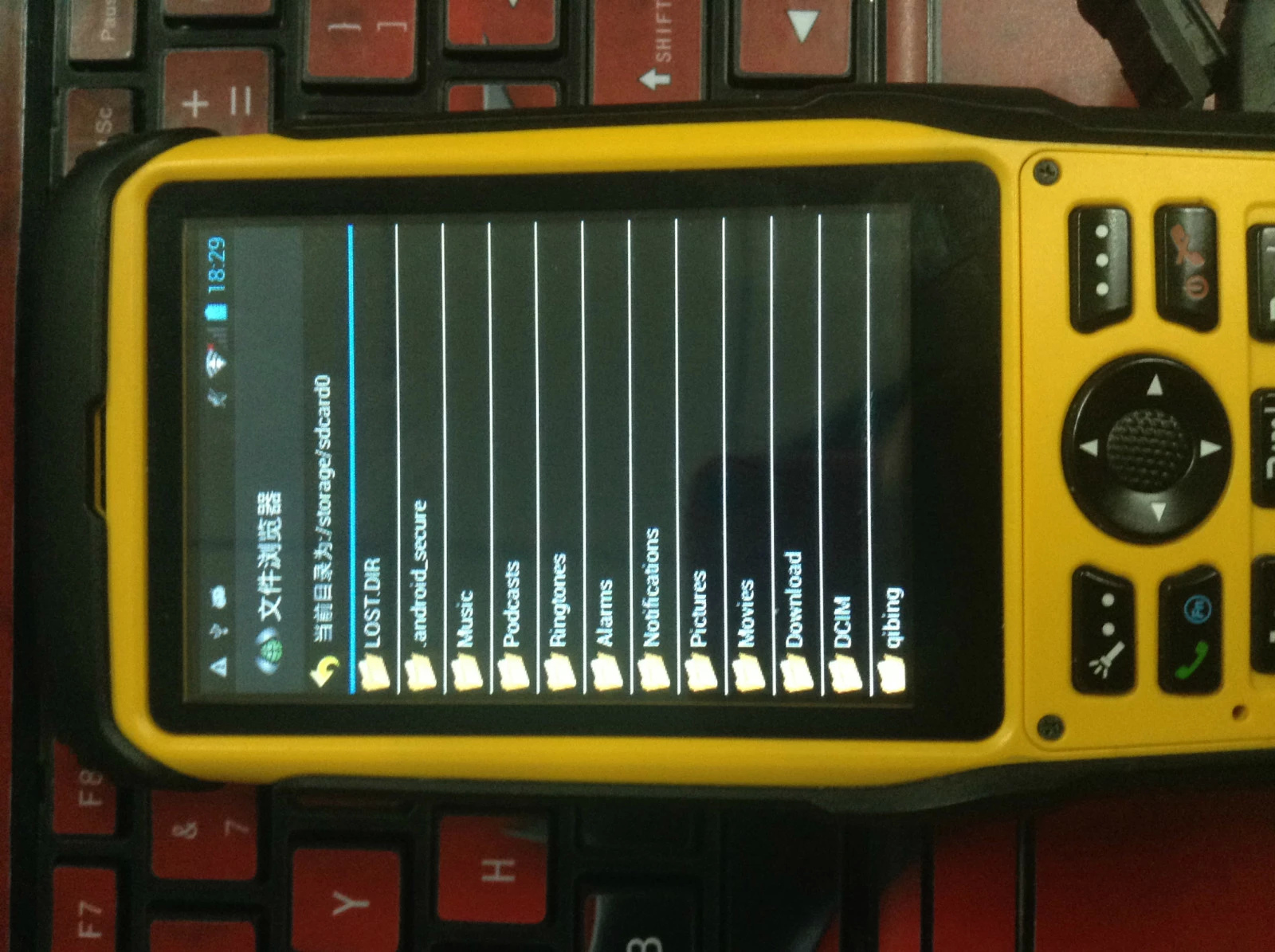
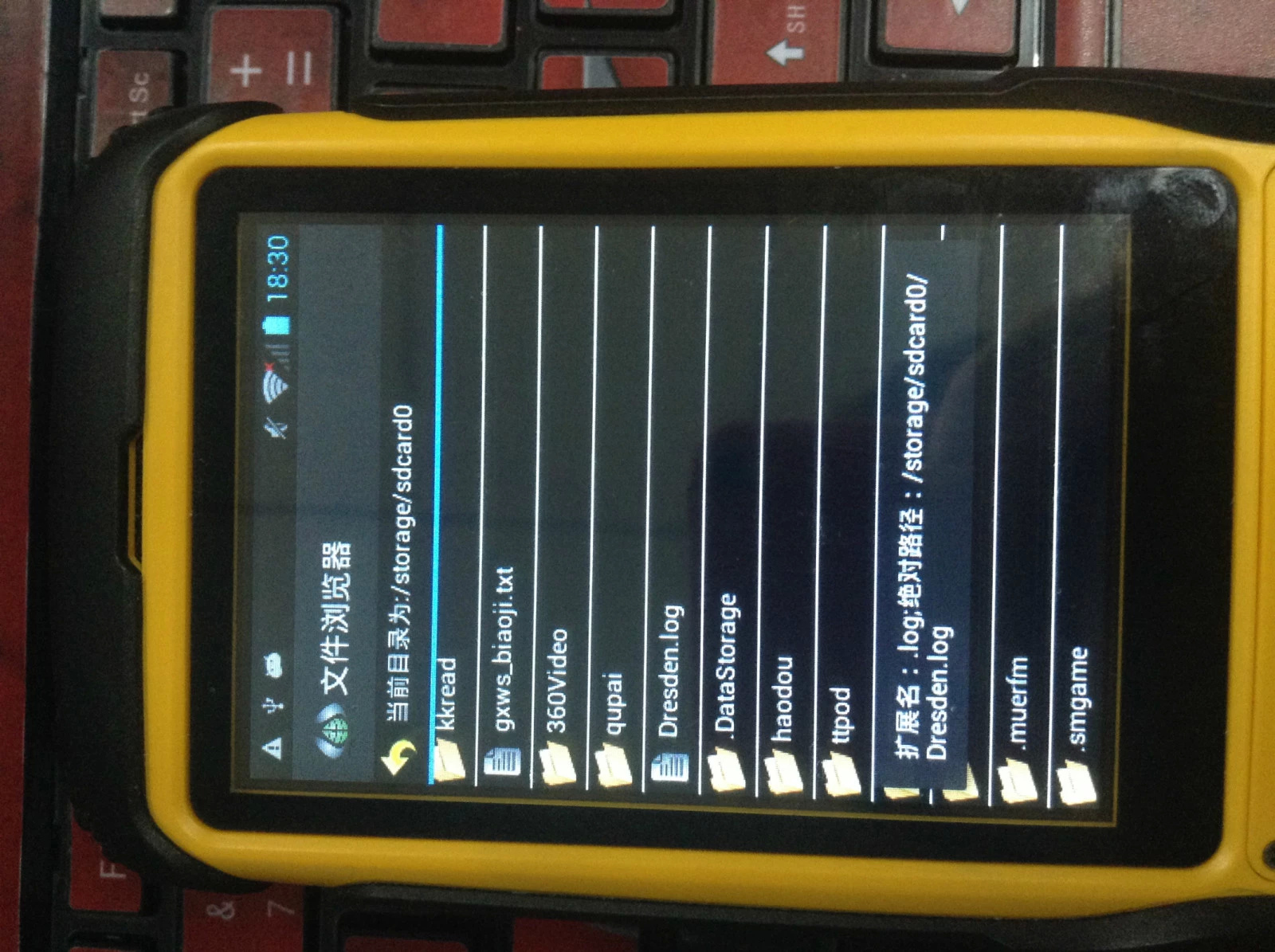
|