本帖最后由 liuzhu 于 2015-9-10 01:18 编辑
gpio general-purpose input/output 通用输入/输出端口
GPIO寄存器缩写列表 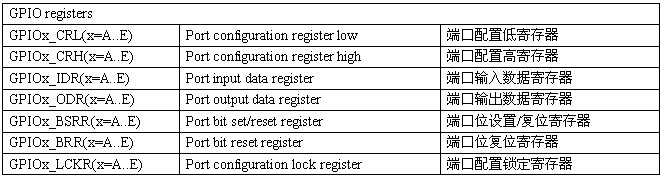 GPIO 端口的每个位可以由软件分别配置成多种模式。 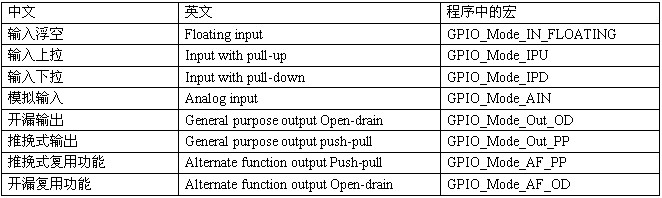
复位期间和刚复位后,复用功能未开启,I/O 端口被配置成浮空输入模式。
LED硬件连接如下图所示:高电平点亮LED。
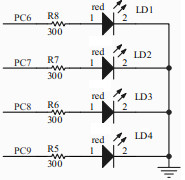
要想成功点亮一个LED,程序所需如下步骤:(必须的) 第一步:配置系统时钟。见STM32F103x RCC寄存器配置
除此之外,还需将GPIO外设时钟打开。
/* Enable GPIOC clock */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
第二步:配置中断向量表。决定将程序下载到RAM中还是FLASH中。以后讲。
void NVIC_Configuration(void)
{
#ifdef VECT_TAB_RAM //VECT_TAB_RAM没在程序中定义,所以将程序下载到Flash中
/* Set the Vector Table base location at 0x20000000 */
NVIC_SetVectorTable(NVIC_VectTab_RAM, 0x0);
#else /* VECT_TAB_FLASH */
/* Set the Vector Table base location at 0x08000000 */
NVIC_SetVectorTable(NVIC_VectTab_FLASH, 0x0);
#endif
}
第三步:配置GPIO的模式。输入模式还是输出模式。本章重点。void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/* Configure PC.06, PC.07, PC.08 and PC.09 as Output push-pull */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7 | GPIO_Pin_8 | GPIO_Pin_9;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOC, &GPIO_InitStructure);
}
其实,使用GPIO十分简单,只需填写如下结构体的成员变量typedef struct
{
u16 GPIO_Pin; //哪个管脚
GPIOSpeed_TypeDef GPIO_Speed; //如果是输出模式的话,还需要设置速度
GPIOMode_TypeDef GPIO_Mode; //管脚的类型
}GPIO_InitTypeDef;
然后,调用GPIO_Init函数,GPIO的模式就配置好了。当然,对于使用者来说,GPIO_Init函数相当于“黑匣子”,我们不知道其内部是怎样实现的,执行完步骤三。我们就可以向该管脚写1还是写0了。提示:GPIO_Init设计的比较巧妙,大家有兴趣的话可以跟踪调试,将该函数中的变量添加到watch窗口,看看GPIO相关寄存器是怎样变化的。第四步:向指定Port指定Pin,写1还是写0。上述原理图中LED都是高电平点亮。需要介绍两个库函数。v GPIO_SetBits 向指定Port指定Pin写1:void GPIO_SetBits(GPIO_TypeDef* GPIOx, u16 GPIO_Pin)
{
/* Check the parameters */
assert_param(IS_GPIO_ALL_PERIPH(GPIOx));
assert_param(IS_GPIO_PIN(GPIO_Pin));
GPIOx->BSRR = GPIO_Pin;
}
涉及到GPIO_BSRR寄存器,如下所示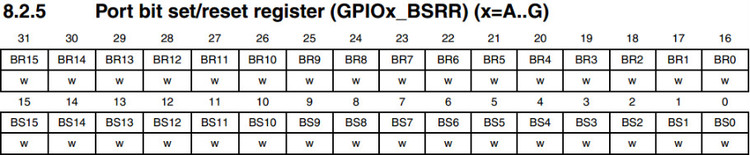
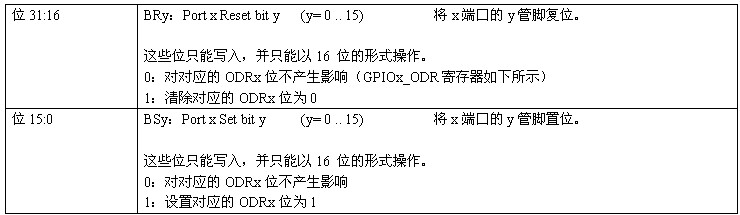
又牵扯到GPIOx_ODR,如下所示 
v GPIO_ResetBits 向指定Port指定Pin写0:
void GPIO_ResetBits(GPIO_TypeDef* GPIOx, u16 GPIO_Pin)
{
/* Check the parameters */
assert_param(IS_GPIO_ALL_PERIPH(GPIOx));
assert_param(IS_GPIO_PIN(GPIO_Pin));
GPIOx->BRR = GPIO_Pin;
}
涉及到GPIO_BRR寄存器,如下所示

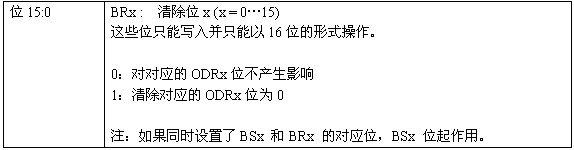
经过上面4步,就可以成功驱动LED。
下面给出LED跑马灯程序:
/* Includes ------------------------------------------------------------------*/
#include "stm32f10x_lib.h"
/* Private function prototypes -----------------------------------------------*/
void RCC_Configuration(void);
void NVIC_Configuration(void);
void GPIO_Configuration(void);
void Delay(vu32 nCount);
/*******************************************************************************
* Function Name : main
* Description : Main program.
* Input : None
* Return : None
*******************************************************************************/
int main(void)
{
#ifdef DEBUG
debug();
#endif
/* Configure the system clocks */
RCC_Configuration();
/* NVIC Configuration */
NVIC_Configuration();
/* Configure the GPIO ports */
GPIO_Configuration();
/* Infinite loop */
while (1)
{
GPIO_SetBits(GPIOC,GPIO_Pin_6);//点亮LED1
Delay(1000000);
Delay(1000000);//多点亮一会,使人能看到LED的确切变化
GPIO_ResetBits(GPIOC,GPIO_Pin_6);//熄灭LED1
GPIO_SetBits(GPIOC,GPIO_Pin_7);//点亮LED2
Delay(1000000);
Delay(1000000);
GPIO_ResetBits(GPIOC,GPIO_Pin_7);//熄灭LED2
GPIO_SetBits(GPIOC,GPIO_Pin_8);//点亮LED3
Delay(1000000);
Delay(1000000);
GPIO_ResetBits(GPIOC,GPIO_Pin_8);//熄灭LED3
GPIO_SetBits(GPIOC,GPIO_Pin_9);//点亮LED4
Delay(1000000);
Delay(1000000);
GPIO_ResetBits(GPIOC,GPIO_Pin_9);//熄灭LED4
}
}
/*******************************************************************************
* Function Name : RCC_Configuration
* Description : Configures the different system clocks.
* Input : None
* Return : None
*******************************************************************************/
void RCC_Configuration(void)
{
ErrorStatus HSEStartUpStatus;
/* RCC system reset(for debug purpose) */
RCC_DeInit();
/* Enable HSE */
RCC_HSEConfig(RCC_HSE_ON);
/* Wait till HSE is ready */
HSEStartUpStatus = RCC_WaitForHSEStartUp();
if (HSEStartUpStatus == SUCCESS)
{
/* Enable Prefetch Buffer */
FLASH_PrefetchBufferCmd(FLASH_PrefetchBuffer_Enable);
/* Flash 2 wait state */
FLASH_SetLatency(FLASH_Latency_2);
/* HCLK = SYSCLK */
RCC_HCLKConfig(RCC_SYSCLK_Div1);
/* PCLK2 = HCLK */
RCC_PCLK2Config(RCC_HCLK_Div1);
/* PCLK1 = HCLK/2 */
RCC_PCLK1Config(RCC_HCLK_Div2);
/* PLLCLK = 8MHz * 9 = 72 MHz */
RCC_PLLConfig(RCC_PLLSource_HSE_Div1, RCC_PLLMul_9);
/* Enable PLL */
RCC_PLLCmd(ENABLE);
/* Wait till PLL is ready */
while(RCC_GetFlagStatus(RCC_FLAG_PLLRDY) == RESET) {}
/* Select PLL as system clock source */
RCC_SYSCLKConfig(RCC_SYSCLKSource_PLLCLK);
/* Wait till PLL is used as system clock source */
while(RCC_GetSYSCLKSource() != 0x08) {}
}
/* Enable GPIOC clock */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
}
/*******************************************************************************
* Function Name : NVIC_Configuration
* Description : Configures Vector Table base location.
* Input : None
* Return : None
*******************************************************************************/
void NVIC_Configuration(void)
{
#ifdef VECT_TAB_RAM
/* Set the Vector Table base location at 0x20000000 */
NVIC_SetVectorTable(NVIC_VectTab_RAM, 0x0);
#else /* VECT_TAB_FLASH */
/* Set the Vector Table base location at 0x08000000 */
NVIC_SetVectorTable(NVIC_VectTab_FLASH, 0x0);
#endif
}
/*******************************************************************************
* Function Name : GPIO_Configuration
* Description : Configures the different GPIO ports.
* Input : None
* Return : None
*******************************************************************************/
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/* Configure PC.06, PC.07, PC.08 and PC.09 as Output push-pull */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7 | GPIO_Pin_8 | GPIO_Pin_9;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOC, &GPIO_InitStructure);
}
/*******************************************************************************
* Function Name : Delay
* Description : Inserts a delay time.
* Input : nCount: specifies the delay time length.
* Return : None
*******************************************************************************/
void Delay(vu32 nCount)
{
for(; nCount != 0; nCount--);
}
#ifdef DEBUG
/*******************************************************************************
* Function Name : assert_failed
* Description : Reports the name of the source file and the source line number
* where the assert_param error has occurred.
* Input : - file: pointer to the source file name
* - line: assert_param error line source number
* Return : None
*******************************************************************************/
void assert_failed(u8* file, u32 line)
{
/* User can add his own implementation to report the file name and line number,
ex: printf("Wrong parameters value: file %s on line %d\r\n", file, line) */
/* Infinite loop */
while (1)
{
}
}
#endif
如何调试:在while (1)处设个断点。 执行完GPIO_Configuration函数后,观察GPIO_CRL和GPIO_CRH寄存器,可以看到: 
每个管脚模式配置由GPIO_CRL或GPIO_CRH中的4位决定,例如:PC6管脚由GPIO_CRL中的MODE6[1:0]和CNF6[1:0]这4位决定,其他的以此类推。 涉及到GPIO_CRL寄存器,如下所示
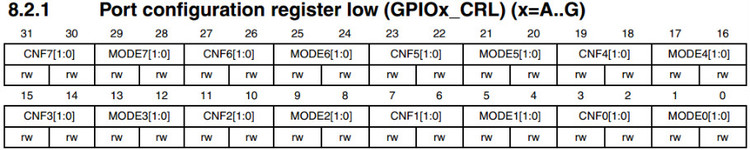 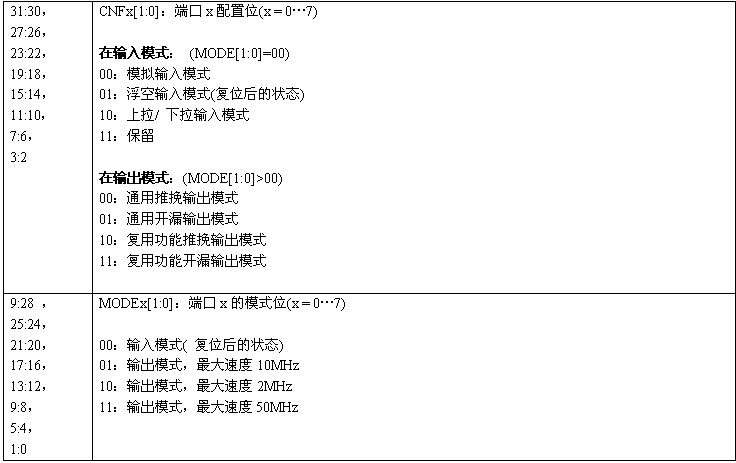
因为MODE6[1:0]=11,查看上述表格,可以得出PC6是输出模式,且最大速度是50MHZ。由于CNF6[1:0]=00且为输出模式,所以以通用推挽输出模式使用输出驱动器。
执行完GPIO_SetBits(GPIOC,GPIO_Pin_6); //点亮LED1,可以看到:GPIO_ODR的ODR6=1 执行完GPIO_ResetBits(GPIOC,GPIO_Pin_6); //熄灭LED1,可以看到:GPIO_ODR的ODR6=0 其他管脚如此类推。
|