一、半加器
Library ieee;
Use ieee.std_logic_1164.all;
Entity halfadd is
Port(a,b:in std_logic;
S,c:out std_logic);
end halfadd;
Architecture add of halfadd is
begin
S<=a xor b;
c<=a and b;
end;
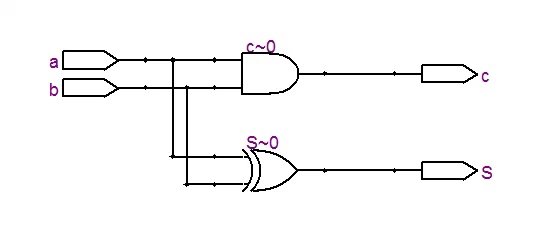
二、全加器
Library ieee;
Use ieee.std_logic_1164.all;
Entity fulladd is
Port(a,b,cin:in std_logic;
S,c:out std_logic);
end fulladd;
Architecture add of fulladd is
signal m,n,k:std_logic;
component halfadd is
Port(a,b:in std_logic;
S,c:out std_logic);
end component;
begin
U0:halfadd port map(a,b,m,n);
U1:halfadd port map(m,cin,S,k);
c<=n or k;
end;
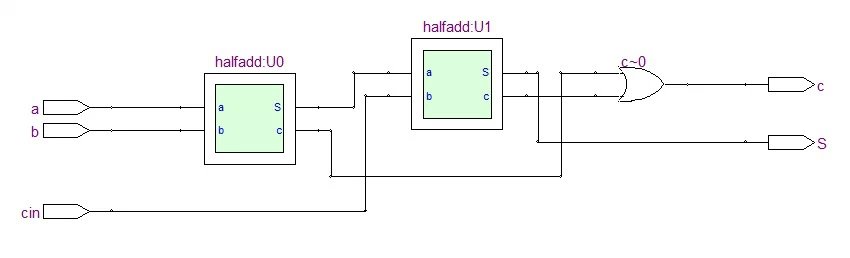
三、四位加法器
一)元件化全加器实现
Library ieee;
Use ieee.std_logic_1164.all;
Entity adder4 is
Port(a,b:in std_logic_vector(3 downto 0);
cin:in std_logic;
S:out std_logic_vector(3 downto 0);
co:out std_logic);
end adder4;
Architecture add of adder4 is
signal c:std_logic_vector(3 downto 0);
component fulladd is
Port(a,b,cin:in std_logic;
S,c:out std_logic);
end component;
begin
U0:fulladd port map(a(0),b(0),cin,S(0),c(0));
U1:fulladd port map(a(1),b(1),c(0),S(1),c(1));
U2:fulladd port map(a(2),b(2),c(1),S(2),c(2));
U3:fulladd port map(a(3),b(3),c(2),S(3),co);
end;
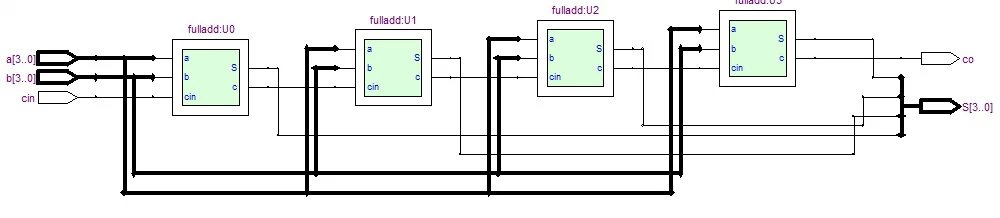
二)循环语句实现
Library ieee;
Use ieee.std_logic_1164.all;
Entity adder4 is
Port(a,b:in std_logic_vector(3 downto 0);
cin:in std_logic;
S:out std_logic_vector(3 downto 0);
co:out std_logic);
end adder4;
Architecture add of adder4 is
begin
Process(a,b,cin)
variable c:std_logic_vector(4 downto 0);
begin
c(0):=cin;
for i in 0 to 3 loop
s(i)<=a(i) xor b(i) xor c(i);
c(i+1):=((a(i) xor b(i))and c(i))or (a(i) and b(i));
end loop;
co<=c(4);
end process;
end;
三)子程序实现
Library ieee;
Use ieee.std_logic_1164.all;
Entity adder4 is
Port(a,b:in std_logic_vector(3 downto 0);
cin:in std_logic;
S:out std_logic_vector(3 downto 0);
co:out std_logic);
end adder4;
Architecture add of adder4 is
Procedure sam(ai,bi,ci:in std_logic;
so,co: out std_logic) is
begin
so:=ai xor bi xor ci;
co:=((ai xor bi)and ci)or(ai and bi);
end sam;
begin
Process(a,b,cin)
variable c:std_logic_vector(4 downto 0);
variable sum:std_logic_vector(3 downto 0);
begin
c(0):=cin;
for i in 0 to 3 loop
sam(a(i),b(i),c(i),Sum(i),c(i+1));
end loop;
co<=c(4);
S<=sum;
end process;
end;
-------n-1位加法器,修改n的值,可以改变运算位数--------
Library ieee;
Use ieee.std_logic_1164.all;
entity adder_n is
generic(n:integer:=4);----改变w的值可以改变运算宽度
port(a,b:in std_logic_vector(n-1 downto 0);
cin:in std_logic;
Sum:out std_logic_vector(n-1 downto 0);
co:out std_logic);
end;
Architecture add of adder_n is
---------------以下子程序---------------------
procedure fulladder(ai,bi,ci:in std_logic;
s,cc:out std_logic)is
begin
s:=ai xor bi xor ci;
cc:=((ai xor bi)and ci)or(ai and bi);
end fulladder;
------------------------------------------
begin
process(a,b,cin)
---loop循环语句必须在process中使用,切子程序要求使用变量,所以在此不使用信号------
variable ss:std_logic_vector(n-1 downto 0);
variable c:std_logic_vector(n downto 0);
-----------------------------------------------------------------------
begin
c(0):=cin;
for i in 0 to n-1 loop
fulladder(a(i),b(i),c(i),ss(i),c(i+1));
end loop;
co<=c(n);
sum<=ss;
end process;
end;
四、八位二进制数相加
一)元件化实现
Library ieee;
Use ieee.std_logic_1164.all;
Entity adder8 is
Port(a,b:in std_logic_vector(7 downto 0);
cin:in std_logic;
S:out std_logic_vector(7 downto 0);
co:out std_logic);
end adder8;
Architecture add of adder8 is
signal a1,a2,b1,b2,s1,s2:std_logic_vector(3 downto 0);
signal cc:std_logic;
component adder4 is
Port(a,b:in std_logic_vector(3 downto 0);
cin:in std_logic;
S:out std_logic_vector(3 downto 0);
co:out std_logic);
end component;
begin
a1<=a(3 downto 0);a2<=a(7 downto 4);
b1<=b(3 downto 0);b2<=b(7 downto 4);
U0:adder4 port map(a1,b1,cin,S1,cc);
U1:adder4 port map(a2,b2,cc,S2,co);
S(7 downto 4)<=S2;S(3 downto 0)<=S1;
end;
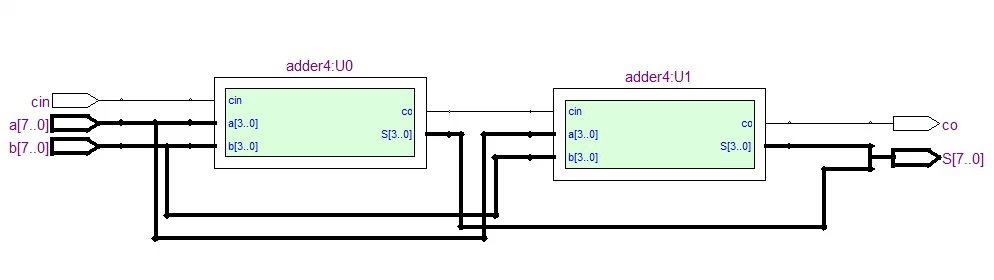
二)循环语句实现
Library ieee;
Use ieee.std_logic_1164.all;
Entity adder4 is
Port(a,b:in std_logic_vector(3 downto 0);
cin:in std_logic;
S:out std_logic_vector(3 downto 0);
co:out std_logic);
end adder4;
Architecture add of adder4 is
variable c:std_logic_vector(8 downto 0);
begin
Process(a,b,cin)
begin
c(0)<=cin;
for i in 0 to 7 loop
s(i)<=a(i) xor b(i) xor c(i);
c(i+1)<=((a(i) xor b(i))and c(i))or (a(i) and b(i));
end loop;
co<=c(8);
end process;
end;
三)子程序实现
Library ieee;
Use ieee.std_logic_1164.all;
Entity adder8 is
Port(a,b:in std_logic_vector(7 downto 0);
cin:in std_logic;
S:out std_logic_vector(7 downto 0);
co:out std_logic);
end adder8;
Architecture add of adder4 is
Procedure sam(ai,bi,ci:in std_logic;
so,co: out std_logic) is
begin
so:=ai xor bi xor ci;
co:=((ai xor bi)and ci)or(ai and bi);
end sam;
begin
Process(a,b,cin)
variable c:std_logic_vector(8 downto 0);
variable sum:std_logic_vector(7 downto 0);
begin
c(0):=cin;
for i in 0 to 7 loop
sam(a(i),b(i),c(i),Sum(i),c(i+1));
end loop;
co<=c(8);
S<=sum;
end process;
end;
减法器接下篇:http://www.51hei.com/bbs/dpj-28501-1.html
|